Navigation & Routing: Integrate deep linking and web features into your Flutter apps.
Introduction
Deep linking and web integration play pivotal roles in enhancing user engagement and accessibility in Flutter apps. As a vital aspect of routing, deep linking enables direct navigation to specific content within an app, while web integration extends app functionality to the browser. In Flutter, routing facilitates seamless navigation between different screens or pages within an app, ensuring a smooth user experience.
Facts about Routing in Flutter:
Flutter's routing system is based on the Navigator class, which manages a stack of Route objects.
Named routes in Flutter provide a convenient way to navigate to specific screens using predefined identifiers.
Flutter's MaterialPageRoute class facilitates transitions between screens with built-in animations and route management functionalities.
Frequently Asked Questions:
What is deep linking, and how does it benefit Flutter apps?
How can Flutter apps leverage web integration for broader reach?
What are the best practices for implementing routing in Flutter?
This blog post is part of the Flutter Learning Roadmap Series, following our previous discussion on "Navigation in Flutter: Learn how to navigate effectively in Flutter apps with tabs, screens, data transfer, and drawers." Stay tuned as we delve deeper into Flutter development, empowering you with the knowledge and skills to build exceptional mobile experiences.
Setting up Deep Linking
Flutter supports deep linking on iOS, Android, and web browsers. Opening a URL displays that screen in your app. With the following steps, you can launch and display routes by using named routes (either with the routes parameter or onGenerateRoute), or by using the Router widget.
Get started:
Refer to the official cookbooks for Android and iOS to get started with deep linking implementation.
Migrating from plugin-based deep linking
If you've previously written a plugin to handle deep links, it will continue to function until you opt in to the new behavior. To do so, add FlutterDeepLinkingEnabled
to Info.plist
for iOS orflutter_deeplinking_enabled
to AndroidManifest.xml
for Android. This enables compatibility with the updated deep linking functionality in Flutter.
Behavior
The behavior of deep linking varies slightly depending on the platform and the app's launch and running state.

iOS (not launched):
Using Navigator: The app gets the initialRoute ("/") and shortly after receives a pushRoute.
Using Router: The app gets the initialRoute ("/") and later uses the RouteInformationParser to parse the route and configure the Navigator with the corresponding Page.
Android - (not launched):
Using Navigator: The app gets the initialRoute containing the route ("/deeplink").
Using Router: Similar to Navigator, the app gets the initialRoute ("/deeplink") and later configures the Navigator with the corresponding Pages.
iOS (launched) and Android (launched):
In both scenarios, pushRoute is called, and the path is parsed. Subsequently, the Navigator is configured with a new set of Pages.
Setting up App Links for Android
Deep linking allows launching an app with a URI, opening it to a specific screen. App links, a type of deep link, are exclusive to Android and use http or https.
1. Customize a Flutter Application:
Utilize the go_router package for routing in your Flutter app. This package simplifies complex routing scenarios and is maintained by the Flutter team. Begin by creating a new Flutter application:
Add the go_router package as a dependency:
2. Modify AndroidManifest.xml:
In the AndroidManifest.xml file, add metadata and intent filters to enable app links. Replace "example.com" with your web domain:
3. Hosting assetlinks.json:
Host an assetlinks.json file on a web server with your domain. This file instructs the mobile browser to open the app instead of the browser. Obtain the package name and sha256 fingerprint of your Flutter app:
Package name: Locate in AndroidManifest.xml under the <manifest> tag.
sha256 fingerprint: Retrieve from Google Play Console or local keystore.
Create the assetlinks.json file:
Testing
Ensure you've run flutter run
at least once on the device to install the app. Then, click the app link URL from a web browser on the device to test. If configured correctly, it should launch the app and navigate to the relevant screen.

To test only the app setup, use the adb command:
To test both web and app setup, you must click a link directly through web browser or another app. One way is to create a Google Doc, add the link, and tap on it.
Set up universal links for iOS
Deep linking enables launching an app with a URI, directing users to specific screens. Universal links are a type of deep link exclusive to Apple devices and utilize http or https.
1. Customize a Flutter Application:
Use the go_router package for routing in your Flutter app. This package simplifies routing scenarios and is maintained by the Flutter team. Create a new Flutter application:
To create a new application.
Add the go_router
package as a dependency:
Create a GoRouter
object in the main.dart
file:
2. Adjust iOS Build Settings:
Launch Xcode and open ios/Runner.xcworkspace.
Navigate to the Info Plist file in ios/Runner.
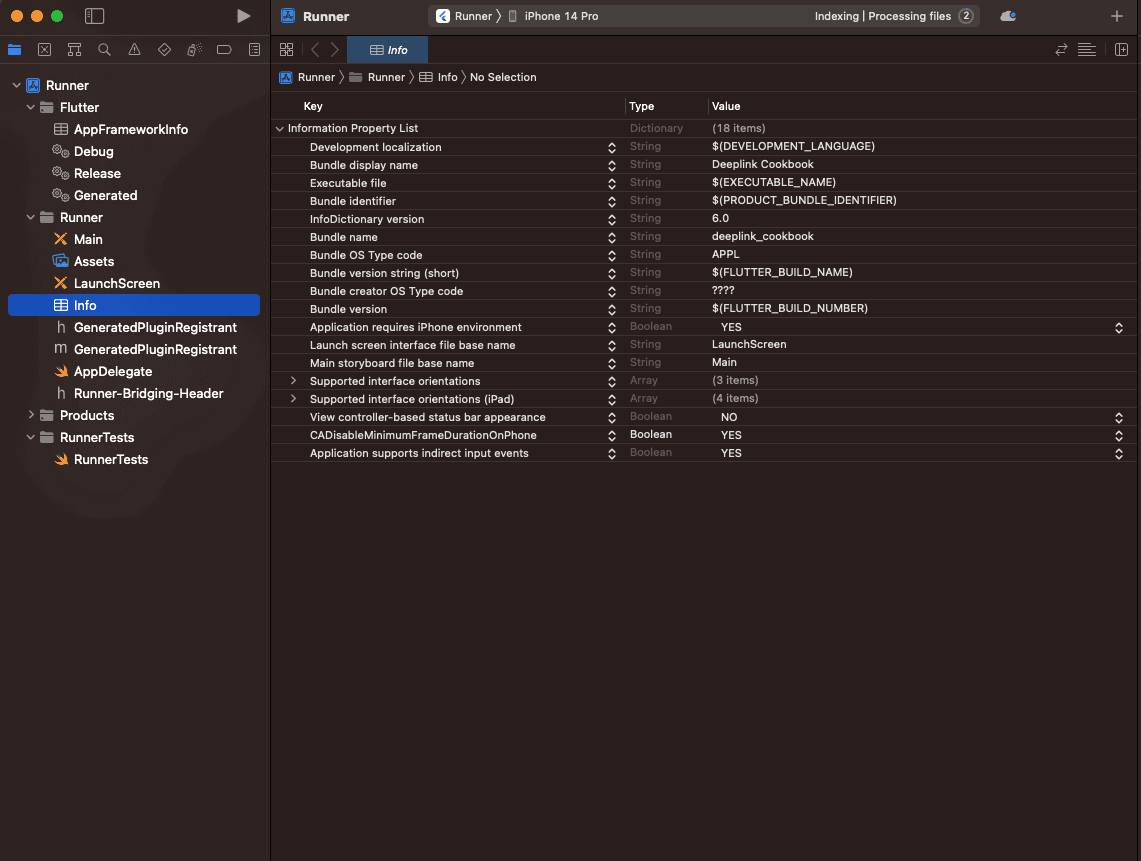
Add FlutterDeepLinkingEnabled with a Boolean value set to YES.

In Signing & Capabilities, add a new domain in Associated Domains.

Enter applinks:<web domain>
. Replace <web domain>
with your own domain name.

3. Hosting apple-app-site-association File:
Host an apple-app-site-association
file on your web domain. This file directs the browser to open the app instead of the browser. Get the app ID by combining the team ID and bundle ID.
The hosted file should contain:
Set appID
to your Flutter app's ID and paths
to ["*"]. Host the file at <webdomain>/.well-known/apple-app-site-association
.
Testing:
Ensure you've run flutter run
at least once on the device. Test the universal link by clicking it on a real device or Simulator. If using the Simulator, test using the Xcode CLI:
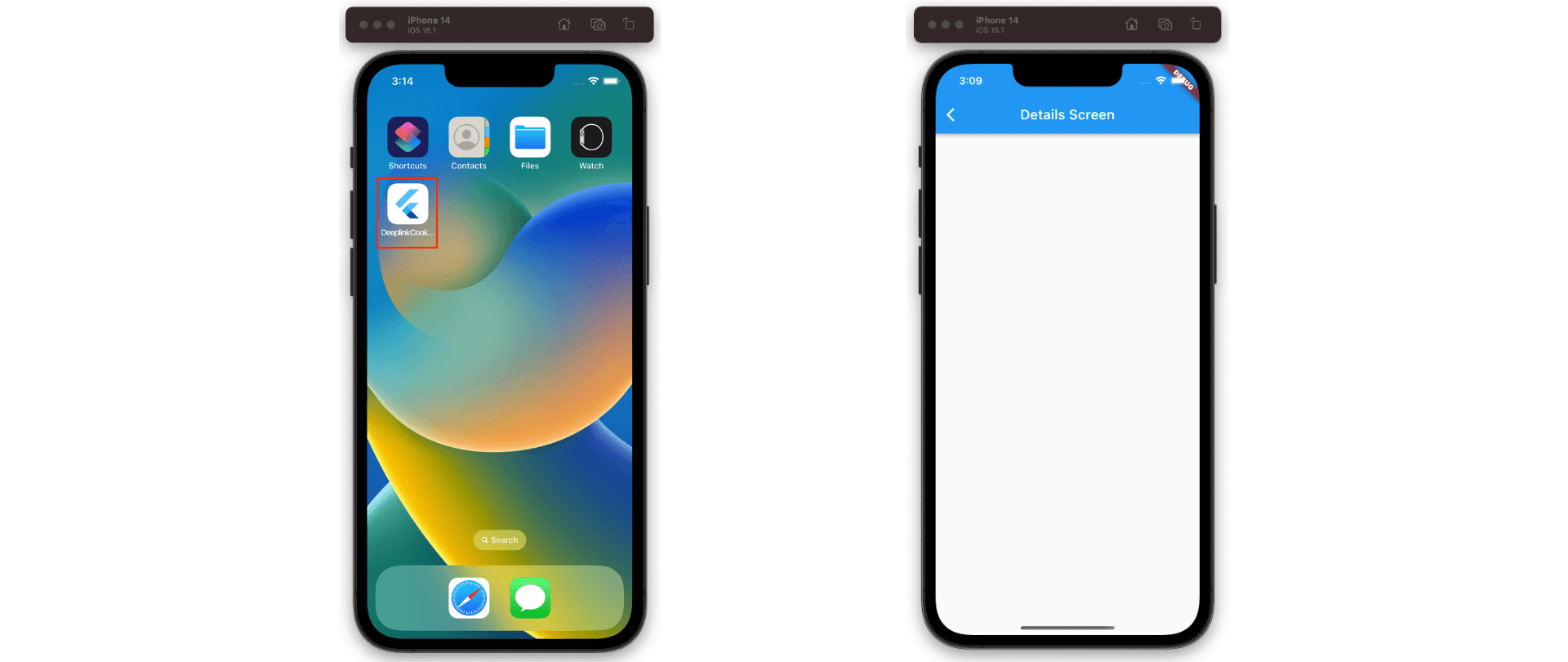
If set up correctly, the Flutter app should launch and display the specified screen.
Configuring URL Navigation in Flutter Web Apps
Flutter web apps support two methods of URL-based navigation:
Hash (Default): Paths are managed using the hash fragment. Example:
flutterexample.dev/#/path/to/screen
.Path: Paths are managed without a hash. Example:
flutterexample.dev/path/to/screen
.
Configuring URL Strategy
To switch Flutter to use the path instead of the hash, utilize the usePathUrlStrategy
function from the flutter_web_plugins
library:
Configuring Web Server
PathUrlStrategy relies on the History API and requires specific web server configuration. Follow your server's documentation to rewrite requests to index.html
. For Firebase Hosting, choose "Configure as a single-page app."
Hosting at a Non-root Location
If hosting your Flutter app at a non-root location, update the <base href="/">
tag in web/index.html
to the app's path. For example, <base href="/flutter_app/">
for hosting at my_app.dev/flutter_app
.
Download Our Flutter-based App Builder "Blup"
Start Building with Blup - Blup's Role in App Development

Unlock the Potential:
Efficiency: Build Flutter apps faster with our intuitive drag-and-drop interface, reducing development time and effort.
Cost-Effective: Save on development costs with BLUP's low-code capabilities, eliminating the need for extensive coding expertise.
Scalability: Seamlessly scale your apps as your business grows, with BLUP's flexible architecture and easy customization options.
Flutter Learning Resources
Explore these recommended resources to deepen your understanding of Flutter navigation:
Official Documentation: Comprehensive guidance and code samples.
Tutorials: Step-by-step guidance on navigation patterns.
Blogs: Insights and tips from Flutter developers.
Videos: Visual tutorials and coding sessions.
Community Forums: Seek help and share knowledge.
Conclusion
In conclusion, we've explored the key concepts of deep linking and web integration in Flutter apps. Deep linking allows for seamless navigation to specific app screens via URIs, enhancing user experience and accessibility. Additionally, integrating web features extends the reach of your app beyond traditional platforms.
By leveraging deep linking and web integration, Flutter apps can provide comprehensive experiences that seamlessly transition between platforms and offer a cohesive user journey. Understanding and implementing these concepts is vital for creating engaging and user-friendly apps in today's digital landscape.